给你两个单链表的头节点 headA
和 headB
,请你找出并返回两个单链表相交的起始节点。如果两个链表没有交点,返回 null
。
图示两个链表在节点 c1
开始相交:
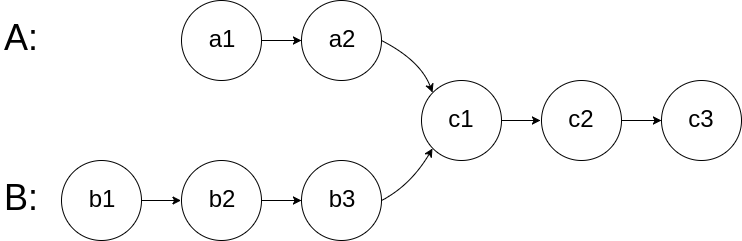
题目数据 保证 整个链式结构中不存在环。
注意,函数返回结果后,链表必须 保持其原始结构 。
示例 1:
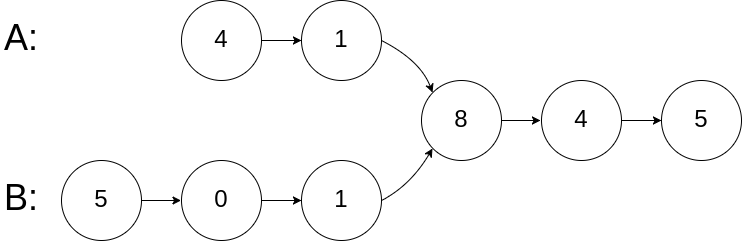
1 2 3 4 5
| 输入:intersectVal = 8, listA = [4,1,8,4,5], listB = [5,0,1,8,4,5], skipA = 2, skipB = 3 输出:Intersected at '8' 解释:相交节点的值为 8 (注意,如果两个链表相交则不能为 0)。 从各自的表头开始算起,链表 A 为 [4,1,8,4,5],链表 B 为 [5,0,1,8,4,5]。 在 A 中,相交节点前有 2 个节点;在 B 中,相交节点前有 3 个节点。
|
示例 2:
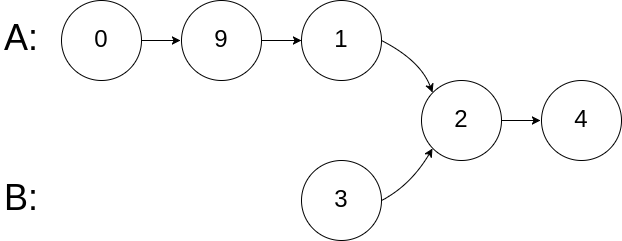
1 2 3 4 5
| 输入:intersectVal = 2, listA = [0,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1 输出:Intersected at '2' 解释:相交节点的值为 2 (注意,如果两个链表相交则不能为 0)。 从各自的表头开始算起,链表 A 为 [0,9,1,2,4],链表 B 为 [3,2,4]。 在 A 中,相交节点前有 3 个节点;在 B 中,相交节点前有 1 个节点。
|
解题思路:
通过一次遍历求出链表长度,记为lenA,lenB
用两个指针分别指向两个链表的头,较长的链表先移动他们差值,假设A比B长,A的指针先移动dis = lenA - lenB次
随后A和B的指针同时移动,第一个遇到的相同的结点,链表相交的起点
若遍历完链表也找不到相同的结点,说明链表不相交,返回null
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| public class Solution { public ListNode getIntersectionNode(ListNode headA, ListNode headB) { ListNode curA = headA; ListNode curB = headB; int lenA = 0, lenB = 0; while(curA != null){ lenA++; curA = curA.next; } while(curB != null){ lenB++; curB = curB.next; } curA = headA; curB = headB; if(lenA > lenB){ int dis = lenA - lenB; while(dis-- != 0){ curA = curA.next; } }else{ int dis = lenB - lenA; while(dis-- != 0){ curB = curB.next; } }
while(curA != null && curB != null){ if(curA == curB){ return curA; } curA = curA.next; curB = curB.next; } return null; } }
|